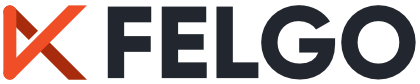
QHttpServer is a simplified API for QAbstractHttpServer and QHttpServerRouter. More...
Header: | #include <QHttpServer> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS HttpServer) target_link_libraries(mytarget PRIVATE Qt6::HttpServer) |
qmake: | QT += httpserver |
Since: | Qt 6.4 |
Inherits: | QAbstractHttpServer |
QHttpServer(QObject *parent = nullptr) | |
virtual | ~QHttpServer() |
void | afterRequest(ViewHandler &&viewHandler) |
bool | route(Args &&... args) |
QHttpServerRouter * | router() |
void | setMissingHandler(QHttpServer::MissingHandler handler) |
QHttpServer server; server.route("/", [] () { return "hello world"; }); server.listen();
[alias]
QHttpServer::MissingHandlerType alias for std::function<void(const QHttpServerRequest &request, QHttpServerResponder &&responder)>.
Creates an instance of QHttpServer with parent parent.
[virtual]
QHttpServer::~QHttpServer()Destroys a QHttpServer.
Register a function to be run after each request.
The viewHandler argument can be a function pointer, non-mutable lambda, or any other copiable callable with const call operator. The callable can take one or two optional arguments: QHttpServerResponse
&&
and const QHttpServerRequest &
. If both are given, they can be in either order.
Examples:
QHttpServer server; // Valid: server.afterRequest([] (QHttpServerResponse &&resp, const QHttpServerRequest &request) { return std::move(resp); } server.afterRequest([] (const QHttpServerRequest &request, QHttpServerResponse &&resp) { return std::move(resp); } server.afterRequest([] (QHttpServerResponse &&resp) { return std::move(resp); } // Invalid (compile time error): // resp must be passed by universal reference server.afterRequest([] (QHttpServerResponse &resp, const QHttpServerRequest &request) { return std::move(resp); } // request must be passed by const reference server.afterRequest([] (QHttpServerResponse &&resp, QHttpServerRequest &request) { return std::move(resp); }
This function is just a wrapper to simplify the router API.
This function takes variadic arguments args. The last argument is a callback (ViewHandler
). The remaining arguments are used to create a new Rule
(the default is QHttpServerRouterRule). This is in turn added to the QHttpServerRouter. It returns true
if a new rule is created, otherwise it returns
false
.
ViewHandler
can be a function pointer, non-mutable lambda, or any other copiable callable with const call operator. The callable can take two optional special arguments: const QHttpServerRequest&
and QHttpServerResponder&&
. These special arguments must be the last in the parameter list, but in any order, and there can be none, one, or both of them present.
Examples:
QHttpServer server; // Valid: server.route("test", [] (const int page) { return ""; }); server.route("test", [] (const int page, const QHttpServerRequest &request) { return ""; }); server.route("test", [] (QHttpServerResponder &&responder) { return ""; }); // Invalid (compile time error): server.route("test", [] (const QHttpServerRequest &request, const int page) { return ""; }); // request must be last server.route("test", [] (QHttpServerRequest &request) { return ""; }); // request must be passed by const reference server.route("test", [] (QHttpServerResponder &responder) { return ""; }); // responder must be passed by universal reference
The request handler may return QFuture<QHttpServerResponse>
if asynchronous processing is desired:
server.route("/feature/", [] (int id) { return QtConcurrent::run([] () { return QHttpServerResponse("the future is coming"); }); });
See also QHttpServerRouter::addRule.
Returns the router object.
Set a handler to call for unhandled paths.
The invocable passed as handler will be invoked for each request that cannot be handled by any of registered route handlers. Passing a default-constructed std::function resets the handler to the default one that produces replies with status 404 Not Found.