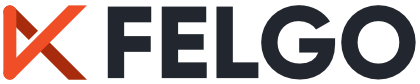
\contentspage
How to make a game like Pong with Felgo - Level Boundaries
First you create a new Wall.qml file in the entities directory. Remove the Rectangle code and add more code which creates an entity, same as the ball before. Now it uses a BoxCollider instead the CircleCollider because the wall element will be rectangular. It consists of two Rectangles in different colors. The second Rectangle is a little bit smaller. The color of the Rectangle is changed when a collision was detected to give visual feedback to the player. Furthermore a timer is started which restores the original color after 500ms.
import QtQuick 2.0 import Felgo 4.0 EntityBase { id: entity entityType: "wall" // Box Collider is used due to the boxed form BoxCollider { id: boxCollider // The wall should not move bodyType: Body.Static // When something collides with the box, this function is called fixture.onBeginContact: { // The color of the inner Rectangle changed to give visual feedback innerRect.color = "green" // start the timer timer.start() } } // When starting the timer it is triggered after 500ms Timer { id: timer interval: 500; onTriggered: { // set the color back to grey innerRect.color = "grey" } } // A Rectangle used as Wall element Rectangle { id: rect color: "black" anchors.fill: parent } // The inner Rectangle is a little bit smaller and changes color when hit by the ball Rectangle { id: innerRect color: "grey" anchors.fill: rect anchors.margins: 0.5 } }
Now you can add some Wall elements to your level. You could add 6 elements on each side. It helps to define a fix width and height for the Walls. Although, the width needs to depend on gameWindowAnchorItem if it should be scalable to support different display sizes.
... // When using the game for several displays it should be anchored correctly anchors.fill: gameWindowAnchorItem property alias ball: ball // 3 Wall blocks for each player side. Use gameWindowAnchorItem for the correct scaling on different display sizes property int blockWidth: gameWindowAnchorItem.width/6 property int blockHeight: 10 ... // The ball started from the middle Ball { id: ball x: gameWindowAnchorItem.width/2 y: parent.height/2 } // Left Player Top Wall { id: partLeftTopP1 height: blockHeight width: blockWidth anchors.left: parent.left anchors.top: parent.top } Wall { id: partMiddleTopP1 height: blockHeight width: blockWidth anchors.left: partLeftTopP1.right anchors.top: parent.top } Wall { id: partRightTopP1 height: blockHeight width: blockWidth anchors.left: partMiddleTopP1.right anchors.top: parent.top } // Left Player Bottom Wall { id: partLeftBottomP1 height: blockHeight width: blockWidth anchors.left: parent.left anchors.bottom: parent.bottom } Wall { id: partMiddleBottomP1 height: blockHeight width: blockWidth anchors.left: partLeftBottomP1.right anchors.bottom: parent.bottom } Wall { id: partRightBottomP1 height: blockHeight width: blockWidth anchors.left: partMiddleBottomP1.right anchors.bottom: parent.bottom } // Right Player Top elements Wall { id: partLeftTopP2 height: blockHeight width: blockWidth anchors.right: partMiddleTopP2.left anchors.top: parent.top } Wall { id: partMiddleTopP2 height: blockHeight width: blockWidth anchors.right: partRightTopP2.left anchors.top: parent.top } Wall { id: partRightTopP2 height: blockHeight width: blockWidth anchors.right: parent.right anchors.top: parent.top } // Right Player Bottom elements Wall { id: partLeftBottomP2 height: blockHeight width: blockWidth anchors.right: partMiddleBottomP2.left anchors.bottom: parent.bottom } Wall { id: partMiddleBottomP2 height: blockHeight width: blockWidth anchors.right: partRightBottomP2.left anchors.bottom: parent.bottom } Wall { id: partRightBottomP2 height: blockHeight width: blockWidth anchors.right: parent.right anchors.bottom: parent.bottom } ...
12 pieces of wall, aligned to provide a barrier on each side where your ball can collide.
Now you wanna add some something to play with, let's continues with paddles.