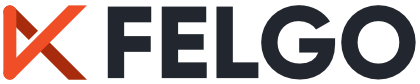
A RayCast object that reports when a ray hits a Fixture. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 2.3.1 |
Inherits: |
The RayCast object can be used to retrieve the fixtureReported signal of a World::rayCast() call.
import QtQuick 2.0 import Felgo 4.0 GameWindow { id: gameWindow screenWidth: 960 screenHeight: 640 Image { anchors.fill: parent source: "background.png" } Scene { id: scene width: 480 height: 320 PhysicsWorld { id: world debugDrawVisible: true gravity.y: 9.81 } // end of PhysicsWorld Repeater { model: 4 WoodenBox { x: Math.random() * (scene.width - 100) + 20 y: Math.random() * (scene.height / 3) + 20 rotation: Math.random() * 90 } } Wall { id: ground height: 20 anchors { left: parent.left; right: parent.right; bottom: parent.bottom } } Wall { id: ceiling height: 20 anchors { left: parent.left; right: parent.right; top: parent.top } } Wall { id: leftWall width: 20 anchors { left: parent.left; bottom: ground.top; top: ceiling.bottom } } Wall { id: rightWall width: 20 anchors { right: parent.right; bottom: ground.top; top: ceiling.bottom } } //show the ray that is being cast as a rectangle Rectangle { property real mx: mouseArea.mouseX - scene.width / 2 property real my: mouseArea.mouseY - scene.height / 2 property real len: 2 * Math.sqrt(mx * mx + my * my) rotation: 180 / Math.PI * Math.atan2(my, mx) transformOrigin: Item.Center x: scene.width / 2 - len / 2 y: scene.height / 2 width: len height: 2 color: "red" } //highlight the object which is hit by the ray Rectangle { id: rect property Item target: null width: target ? target.width : 0 height: target ? target.height : 0 x: target ? target.x : 0 y: target ? target.y : 0 color: "green" opacity: 0.5 rotation: target ? target.rotation : 0 visible: !!target transformOrigin: Item.TopLeft } RayCast { id: raycast maxFraction: 2 //cast ray twice the distance from start to end point onFixtureReported: (fixture, contactPoint, contactNormal, fraction) => { //fixture.getBody() returns the body object, body.target returns the entity which contains the body var body = fixture.getBody() var entity = body.target rect.target = entity maxFraction = 0 // cancel current raycast, report no more objects } } MouseArea { id: mouseArea anchors.fill: parent hoverEnabled: true onClicked: { rect.target = null raycast.maxFraction = 2 //cast a ray from the mouse position through the center of the scene var from = Qt.point(mouse.x, mouse.y) var to = Qt.point(scene.width / 2, scene.height / 2) console.log("raycast", from, to) world.rayCast(raycast, from, to) } } } // end of Scene EntityManager { id: entityManager } }
See the folder examples/physics/box2d-examples/qml/raycast
for the Wall and WoodenBox implementation and for the image resources.
maxFraction : real |
This property determines length of the cast ray, as fraction of the length between the points from and to. After fixtureReported is called, it gets set to the length of the ray where it hit the fixture. You can then change the value of maxFraction to a larger value, to query for more fixtures, or to a lower value to stop querying.
The default value is -1, which means default length between points from and to.
This handler is called when the ray hits a Fixture after casting it with World::rayCast().
You can retrieve information of the collided fixture with the fixture argument. The contactPoint tells where the fixture has been hit, the contactNormal is the normal on the fixture.
The argument fraction tells the length of the ray, as a fraction of the length between the start and end point. This may also be greater than 1, if the maxFraction field has been set accordingly.
To get access to the collided entity, use this example code:
import Felgo 4.0 RayCast { id: raycast maxFraction: 2 //cast ray twice the distance from start to end point onFixtureReported: (fixture, contactPoint, contactNormal, fraction) => { console.debug("fixtureReported:", fixture) //fixture.getBody() returns the body object, body.target returns the entity which contains the body var body = fixture.getBody() var entity = body.target maxFraction = 0 // cancel current raycast, report no more objects } }
Note: The corresponding handler is onFixtureReported
.